Upload a File and Send Using Node Js
Editor's note: This article was terminal updated 24 March 2022 to reflect updates to Node.js and the body-parser
library.
Multer is a Node.js middleware for treatment multipart/class-data
that makes the otherwise painstaking process of uploading files in Node.js much easier. In this article, we'll learn the purpose of Multer in handling files in submitted forms. We'll as well explore Multer by building a mini app with a frontend and backend to test uploading a file. Let'due south get started!
Table of contents
- Managing user inputs in forms
- Encoding and uploading forms with Multer
- Multer: an overview
- Building an app with Multer support
- Creating our frontend
- Install and configure Multer
- Conclusion
Managing user inputs in forms
Web applications receive all different types of input from users, including text, graphical controls like checkboxes or radio buttons, and files, like images, videos, and other media.
In forms, each of these inputs are submitted to a server that processes the inputs, uses them in some mode, perhaps saving them somewhere else, then gives the frontend a success
or failed
response.
When submitting forms that comprise text inputs, the server, Node.js in our instance, has less work to do. Using Express, you tin easily grab all the inputs entered in the req.body
object. Yet, submitting forms with files is a bit more circuitous because they require more than processing, which is where Multer comes in.
Encoding and uploading forms with Multer
All forms include an enctype
attribute, which specifies how data should be encoded by the browser before sending it to the server. The default value is application/x-www-form-urlencoded
, which supports alphanumeric data. The other encoding blazon is multipart/grade-data
, which involves uploading files through forms.
At that place are two ways to upload forms with multipart/form-data
encoding. The outset is by using the enctype
attribute:
<form action='/upload_files' enctype='multipart/form-data'> ... </form>
The code above sends the form-information to the /upload_files
path of your application. The 2nd is by using the FormData
API. The FormData
API allows us to build a multipart/form-data
form with key-value pairs that can be sent to the server. Here's how it's used:
const class = new FormData() form.append('name', "Dillion") class.append('image', <a file>)
On sending such forms, it becomes the server's responsibility to correctly parse the form and execute the final operation on the information.
Multer: an overview
Multer is a middleware designed to handle multipart/form-data
in forms. It is like to the popular Node.js body-parser
, which is built into Express middleware for class submissions. But, Multer differs in that it supports multipart information, only processing multipart/form-data
forms.
Multer does the work of trunk-parser
by attaching the values of text fields in the req.body
object. Multer too creates a new object for multiple files, eitherreq.file
or req.files
, which holds information about those files. From the file object, you tin can pick whatever information is required to post the file to a media management API, similar Cloudinary.
Now that we understand the importance of Multer, we'll build a small sample app to evidence how a frontend app can transport iii different files at in one case in a class, and how Multer is able to procedure the files on the backend, making them available for further apply.
Building an app with Multer support
Nosotros'll start past building the frontend using vanilla HTML, CSS, and JavaScript. Of course, you lot tin can easily apply any framework to follow forth.
Creating our frontend
Start, create a folder called file-upload-example
, then create another binder called frontend
inside. In the frontend folder, we'll have three standard files, index.html
, styles.css
, and script.js
:
<!-- index.html --> <body> <div class="container"> <h1>File Upload</h1> <class id='form'> <div class="input-group"> <label for='proper noun'>Your proper name</label> <input proper name='name' id='name' placeholder="Enter your proper name" /> </div> <div class="input-group"> <label for='files'>Select files</label> <input id='files' type="file" multiple> </div> <button class="submit-btn" blazon='submit'>Upload</push button> </form> </div> <script src='./script.js'></script> </body>
Notice that we've created a label and input for Your Name
equally well as Select Files
. We besides added an Upload
button.
Next, we'll add the CSS for styling:
/* style.css */ torso { groundwork-color: rgb(6, 26, 27); } * { box-sizing: border-box; } .container { max-width: 500px; margin: 60px motorcar; } .container h1 { text-align: middle; color: white; } form { background-colour: white; padding: 30px; } course .input-group { margin-lesser: 15px; } class label { brandish: block; margin-bottom: 10px; } form input { padding: 12px 20px; width: 100%; border: 1px solid #ccc; } .submit-btn { width: 100%; border: none; groundwork: rgb(37, 83, 3); font-size: 18px; color: white; border-radius: 3px; padding: 20px; text-align: center; }
Below is a screenshot of the webpage so far:
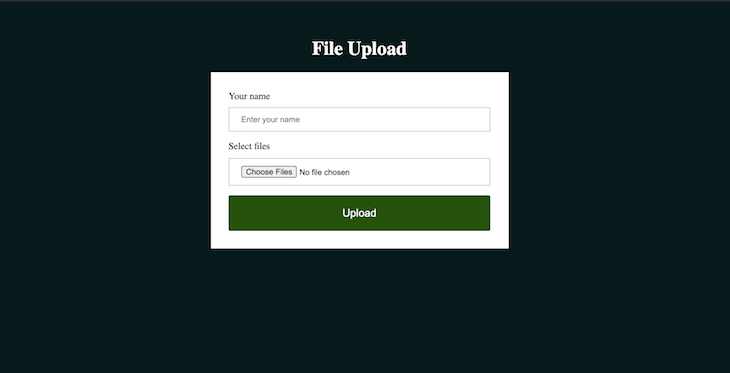
As you can see, the form we created takes two inputs, name
and files
. The multiple
attribute specified in the files
input enables u.s.a. to select multiple files.
Next, we'll send the form to the server using the lawmaking below:
// script.js const form = document.getElementById("grade"); class.addEventListener("submit", submitForm); function submitForm(e) { e.preventDefault(); const proper noun = document.getElementById("proper noun"); const files = document.getElementById("files"); const formData = new FormData(); formData.append("proper name", name.value); for(allow i =0; i < files.files.length; i++) { formData.suspend("files", files.files[i]); } fetch("http://localhost:5000/upload_files", { method: 'Mail', torso: formData, headers: { "Content-Type": "multipart/form-information" } }) .and then((res) => console.log(res)) .catch((err) => ("Error occured", err)); }
There are several important things that must happen when we utilize script.js
. Showtime, nosotros go the form
element from the DOM and add a submit
issue to it. Upon submitting, we use preventDefault
to preclude the default activity that the browser would have when a class is submitted, which would normally be redirecting to the value of the action
attribute. Next, nosotros go the name
and files
input element from the DOM and createformData.
From hither, we'll suspend the value of the name input using a cardinal of name
to the formData
. So, nosotros dynamically add the multiple files we selected to the formData
using a key of files
.
Note: if we're simply concerned with a single file, nosotros can append
files.files[0]
.
Finally, nosotros'll add a Mail service
asking to http://localhost:5000/upload_files
, which is the API on the backend that nosotros'll build in the next section.
Setting up the server
For our demo, we'll build our backend using Node.js and Express. We'll set up upward a simple API in upload_files
and outset our server on localhost:5000
. The API will receive a POST
request that contains the inputs from the submitted form.
To utilize Node.js for our server, we'll demand to ready a basic Node.js project. In the root directory of the projection in the terminal at file-upload-example
, run the post-obit code:
npm init -y
The command above creates a basic package.json
with some information about your app. Adjacent, we'll install the required dependency, which for our purposes is Express:
npm i express
Next, create a server.js
file and add the post-obit code:
// server.js const express = require("express"); const app = express(); app.use(express.json()); app.use(express.urlencoded({ extended: true })); app.postal service("/upload_files", uploadFiles); role uploadFiles(req, res) { panel.log(req.trunk); } app.listen(5000, () => { console.log(`Server started...`); });
Express contains the bodyParser
object, which is a middleware for populating req.body
with the submitted inputs on a form. Calling app.use(express.json())
executes the middleware on every request made to our server.
The API is set up with app.post('/upload_files', uploadFiles)
. uploadFiles
is the API controller. As seen above, nosotros are merely logging out req.trunk
, which should be populated past epxress.json()
. We'll test this out in the example below.
Running body-parser
in Limited
In your terminal, run node server
to start the server. If done correctly, you'll run into the post-obit in your terminal:
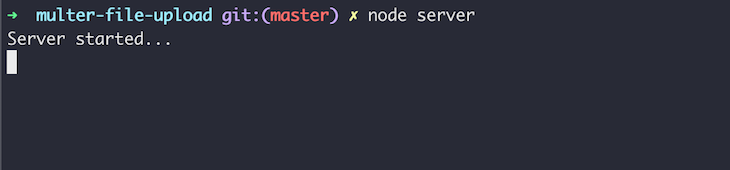
Y'all tin now open your frontend app in your browser. Fill in both inputs in the frontend, the name and files, then click submit. On your backend, yous should see the post-obit:
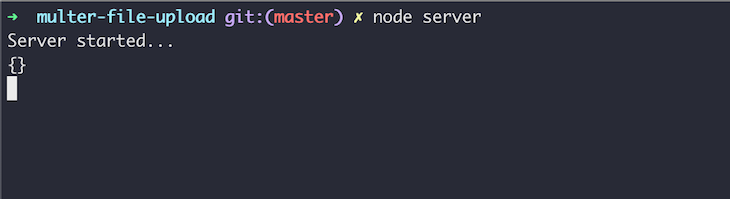
The code in the image in a higher place ways that the req.trunk
object is empty, which is to exist expected. If you lot'll recollect, torso-parser
doesn't support multipart
information. Instead, we'll employ Multer to parse the form.
Install and configure Multer
Install Multer by running the post-obit control in your terminal:
npm i multer
To configure Multer, add the following to the top of server.js
:
const multer = require("multer"); const upload = multer({ dest: "uploads/" }); ...
Although Multer has many other configuration options, nosotros're only interested in thedest
property for our project, which specifies the directory where Multer will relieve the encoded files.
Side by side, nosotros'll use Multer to intercept incoming requests on our API and parse the inputs to make them available on the req
object:
app.mail service("/upload_files", upload.array("files"), uploadFiles); function uploadFiles(req, res) { console.log(req.body); panel.log(req.files); res.json({ bulletin: "Successfully uploaded files" }); }
To handle multiple files, employ upload.assortment
. For a single file, use upload.unmarried
. Note that the files
statement depends on the proper noun of the input specified in formData
.
Multer will add the text inputs to req.torso
and add together the files sent to the req.files
array. To see this at piece of work in the concluding, enter text and select multiple images on the frontend, then submit and check the logged results in your terminal.
Every bit you can see in the example beneath, I entered Images
in the text
input and selected a PDF, an SVG, and a JPEG file. Below is a screenshot of the logged issue:
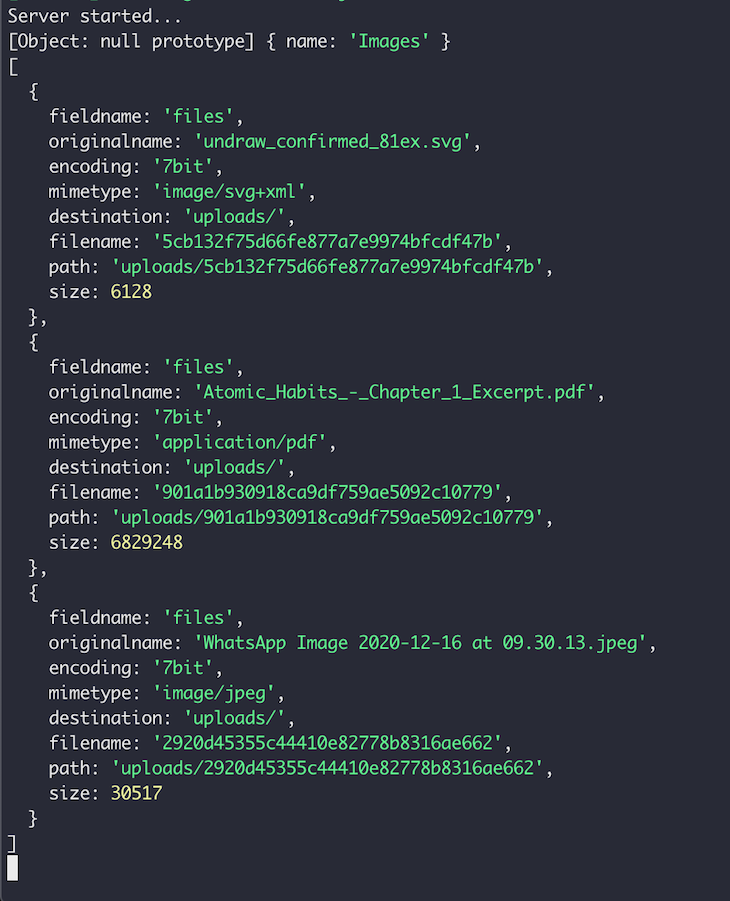
For reference, if you want to upload to a storage service like Cloudinary, you will accept take to ship the file directly from the uploads folder. The path
property shows the path to the file.
Conclusion
For text inputs alone, the bodyParser
object used inside of Limited is enough to parse those inputs. They make the inputs available as a key value pair in the req.body
object. Multer comes in handy when forms contain multipart
data that includes text inputs and files, which the body-parser
library cannot handle.
With Multer, you can handle unmarried or multiple files in addition to text inputs sent through a class. Recall that you lot should only use Multer when you're sending files through forms, because Multer cannot handle any form that isn't multipart.
In this article, we've seen a brief of grade submissions, the benefits of body parsers on the server and the role that Multer plays in treatment form inputs. We also built a modest awarding using Node.js and Multer to run into a file upload process.
For the side by side steps, you tin await at uploading to Cloudinary from your server using the Upload API Reference. I hope y'all enjoyed this article! Happy coding!
200's just
Monitor failed and dull network requests in production
Deploying a Node-based web app or website is the piece of cake part. Making sure your Node instance continues to serve resources to your app is where things get tougher. If yous're interested in ensuring requests to the backend or 3rd party services are successful, try LogRocket. https://logrocket.com/signup/
LogRocket is like a DVR for web and mobile apps, recording literally everything that happens while a user interacts with your app. Instead of guessing why issues happen, you lot can aggregate and report on problematic network requests to quickly sympathize the root cause.
LogRocket instruments your app to record baseline functioning timings such as page load fourth dimension, time to first byte, slow network requests, and likewise logs Redux, NgRx, and Vuex actions/country. Showtime monitoring for free.
Source: https://blog.logrocket.com/multer-nodejs-express-upload-file/
0 Response to "Upload a File and Send Using Node Js"
إرسال تعليق